Here's the result:
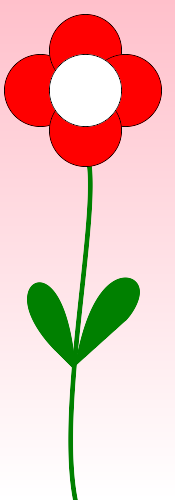
<?php
class Flower extends ImagickDraw
{
private $ImagickPixel;
public function __construct()
{
$this->ImagickPixel = new ImagickPixel();
}
public function drawFlower()
{
$this->createStraw();
$this->createPetals();
$this->createLeafs();
}
private function createPetals()
{
$this->ImagickPixel->setColor( 'red' );
$this->setFillColor( $this->ImagickPixel );
$this->ImagickPixel->setColor( 'black' );
$this->setStrokeColor( $this->ImagickPixel );
$this->setStrokeWidth( 0.5 );
$this->circle( 40, 90, 70, 110 );
$this->circle( 85, 50, 115, 70 );
$this->circle( 125, 90, 155, 110 );
$this->circle( 85, 130, 115, 150 );
$this->ImagickPixel->setColor( 'white' );
$this->setFillColor( $this->ImagickPixel );
$this->circle( 85, 90, 115, 110 );
}
private function createStraw()
{
$this->ImagickPixel->setColor( 'transparent' );
$this->setFillColor( $this->ImagickPixel );
$this->ImagickPixel->setColor( 'green' );
$this->setStrokeColor( $this->ImagickPixel );
$this->setStrokeWidth( 4 );
$this->bezier( array(
array( 'x' => 85, 'y' => 150 ),
array( 'x' => 105, 'y' => 190 ),
array( 'x' => 55, 'y' => 400 ),
array( 'x' => 75, 'y' => 500 ),
)
);
}
private function createLeafs()
{
$this->ImagickPixel->setColor( 'green' );
$this->setFillColor( $this->ImagickPixel );
$this->setStrokeColor( $this->ImagickPixel );
$this->bezier( array(
array( 'x' => 75, 'y' => 370 ),
array( 'x' => 65, 'y' => 250 ),
array( 'x' => 25, 'y' => 270 ),
array( 'x' => 15, 'y' => 290 ),
array( 'x' => 25, 'y' => 310 ),
array( 'x' => 35, 'y' => 330 ),
array( 'x' => 55, 'y' => 350 ),
)
);
$this->bezier( array(
array( 'x' => 75, 'y' => 365 ),
array( 'x' => 95, 'y' => 250 ),
array( 'x' => 135, 'y' => 270 ),
array( 'x' => 145, 'y' => 280 ),
array( 'x' => 145, 'y' => 290 ),
array( 'x' => 135, 'y' => 310 ),
array( 'x' => 125, 'y' => 320 ),
)
);
}
}
$Flower = new Flower();
$Flower->drawFlower();
$Imagick = new Imagick();
$Imagick->newPseudoImage( 175, 500, "gradient:pink-white" );
$Imagick->setImageFormat( 'png' );
$Imagick->drawImage( $Flower );
header( "Content-Type: image/{$Imagick->getImageFormat()}" );
echo $Imagick;
?>
|