This is how the resulting image looks like:
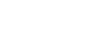
<?php
/* This object will hold the animation */
$animation = new Imagick();
/* Set the format to gif. All created images will be gifs*/
$animation->setFormat( "gif" );
/* Create a pixel to handle colors */
$color = new ImagickPixel( "white" );
/* The first frame */
$color->setColor( "white" );
/* This is the string we print on the image
Character by character */
$string = "HELLO WORLD!";
/* Create a new ImagickDraw object and set the font
Text color defaults to black */
$draw = new ImagickDraw();
/* You can get configured fonts with $im->queryFonts
or use /path/to/file.ttf */
$draw->setFont( "Helvetica" );
/* Loop trough the string.. */
for ( $i = 0; $i <= strlen( $string ); $i++ )
{
/* Take some characters for this frame */
$part = substr( $string, 0, $i );
/* Create a new frame */
$animation->newImage( 100, 50, $color );
/* Annotate the characters on the image */
$animation->annotateImage( $draw, 10, 10, 0, $part );
/* Set a little higher delay for the frame*/
$animation->setImageDelay( 30 );
}
/* The last frame contains the same text with bold */
$draw->setFont( "Helvetica-Bold" );
/* One more frame */
$animation->newImage( 100, 50, $color );
/* Annotate the bold text on the image */
$animation->annotateImage( $draw, 10, 10, 0, $string );
/* The bold can be visible a little longer time */
$animation->setImageDelay( 60 );
/* Display the output as an animation.
Use getImagesBlob to get all images combined */
header( "Content-Type: image/gif" );
echo $animation->getImagesBlob();
?>
|