The reflection is created by flipping the image and overlaying a gradient on it. Then both, the original image and the reflection is overlayed on a canvas.
This example is created for Imagick 2.1.x but with a little tuning it should work with earlier versions.
The source image:
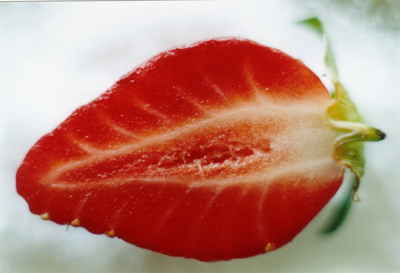
And the result:
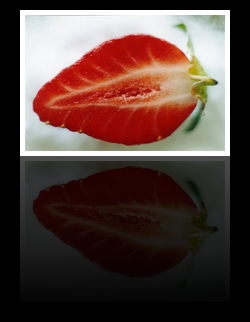
<?php
/* Read the image */
$im = new Imagick( "strawberry.png" );
/* Thumbnail the image */
$im->thumbnailImage( 200, null );
/* Create a border for the image */
$im->borderImage( "white", 5, 5 );
/* Clone the image and flip it */
$reflection = $im->clone();
$reflection->flipImage();
/* Create gradient. It will be overlayd on the reflection */
$gradient = new Imagick();
/* Gradient needs to be large enough for the image
and the borders */
$gradient->newPseudoImage( $reflection->getImageWidth() + 10,
$reflection->getImageHeight() + 10,
"gradient:transparent-black"
);
/* Composite the gradient on the reflection */
$reflection->compositeImage( $gradient, imagick::COMPOSITE_OVER, 0, 0 );
/* Add some opacity */
$reflection->setImageOpacity( 0.3 );
/* Create empty canvas */
$canvas = new Imagick();
/* Canvas needs to be large enough to hold the both images */
$width = $im->getImageWidth() + 40;
$height = ( $im->getImageHeight() * 2 ) + 30;
$canvas->newImage( $width, $height, "black", "png" );
/* Composite the original image and the reflection on the canvas */
$canvas->compositeImage( $im, imagick::COMPOSITE_OVER, 20, 10 );
$canvas->compositeImage( $reflection, imagick::COMPOSITE_OVER,
20, $im->getImageHeight() + 10 );
/* Output the image*/
header( "Content-Type: image/png" );
echo $canvas;
?>
|